mirror of
https://github.com/mcMMO-Dev/mcMMO.git
synced 2025-04-03 02:06:23 +02:00
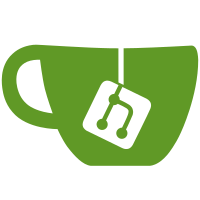
Fixed a glitch that let players remove the ingredient of a custom potion from the brewing stand without cancelling the brewing task (this doesn't seem to have caused any duplicates, once the task finished nothing happened).
41 lines
1.6 KiB
Java
41 lines
1.6 KiB
Java
package com.gmail.nossr50.runnables.skills;
|
|
|
|
import java.util.Arrays;
|
|
|
|
import org.bukkit.Location;
|
|
import org.bukkit.block.BrewingStand;
|
|
import org.bukkit.entity.Player;
|
|
import org.bukkit.inventory.ItemStack;
|
|
import org.bukkit.scheduler.BukkitRunnable;
|
|
|
|
import com.gmail.nossr50.skills.alchemy.Alchemy;
|
|
import com.gmail.nossr50.skills.alchemy.AlchemyPotionBrewer;
|
|
|
|
public class AlchemyBrewCheckTask extends BukkitRunnable {
|
|
private Player player;
|
|
private BrewingStand brewingStand;
|
|
private ItemStack[] oldInventory;
|
|
|
|
public AlchemyBrewCheckTask(Player player, BrewingStand brewingStand) {
|
|
this.player = player;
|
|
this.brewingStand = brewingStand;
|
|
this.oldInventory = Arrays.copyOfRange(brewingStand.getInventory().getContents(), 0, 4);
|
|
}
|
|
|
|
@Override
|
|
public void run() {
|
|
Location location = brewingStand.getLocation();
|
|
ItemStack[] newInventory = Arrays.copyOfRange(brewingStand.getInventory().getContents(), 0, 4);
|
|
boolean validBrew = brewingStand.getFuelLevel() > 0 && AlchemyPotionBrewer.isValidBrew(player, newInventory);
|
|
|
|
if (Alchemy.brewingStandMap.containsKey(location)) {
|
|
if (oldInventory[Alchemy.INGREDIENT_SLOT] == null || newInventory[Alchemy.INGREDIENT_SLOT] == null || !oldInventory[Alchemy.INGREDIENT_SLOT].isSimilar(newInventory[Alchemy.INGREDIENT_SLOT]) || !validBrew) {
|
|
Alchemy.brewingStandMap.get(location).cancelBrew();
|
|
}
|
|
}
|
|
else if (validBrew) {
|
|
Alchemy.brewingStandMap.put(location, new AlchemyBrewTask(brewingStand, player));
|
|
}
|
|
}
|
|
}
|