mirror of
https://github.com/mcMMO-Dev/mcMMO.git
synced 2024-10-17 17:43:39 +02:00
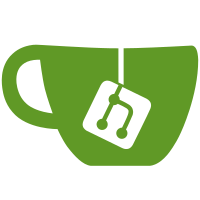
This will make sure no experience is lost when the server has high skill modifiers configured, or when low amounts of experience are being shared.
45 lines
1.2 KiB
Java
45 lines
1.2 KiB
Java
package com.gmail.nossr50.skills;
|
|
|
|
import org.bukkit.entity.Player;
|
|
|
|
import com.gmail.nossr50.datatypes.player.McMMOPlayer;
|
|
import com.gmail.nossr50.datatypes.player.PlayerProfile;
|
|
import com.gmail.nossr50.datatypes.skills.SkillType;
|
|
import com.gmail.nossr50.util.skills.PerksUtils;
|
|
|
|
public abstract class SkillManager {
|
|
protected McMMOPlayer mcMMOPlayer;
|
|
protected int activationChance;
|
|
protected SkillType skill;
|
|
|
|
public SkillManager(McMMOPlayer mcMMOPlayer, SkillType skill) {
|
|
this.mcMMOPlayer = mcMMOPlayer;
|
|
this.activationChance = PerksUtils.handleLuckyPerks(mcMMOPlayer.getPlayer(), skill);
|
|
this.skill = skill;
|
|
}
|
|
|
|
public McMMOPlayer getMcMMOPlayer() {
|
|
return mcMMOPlayer;
|
|
}
|
|
|
|
public Player getPlayer() {
|
|
return mcMMOPlayer.getPlayer();
|
|
}
|
|
|
|
public PlayerProfile getProfile() {
|
|
return mcMMOPlayer.getProfile();
|
|
}
|
|
|
|
public int getSkillLevel() {
|
|
return mcMMOPlayer.getProfile().getSkillLevel(skill);
|
|
}
|
|
|
|
public int getActivationChance() {
|
|
return activationChance;
|
|
}
|
|
|
|
public void applyXpGain(float xp) {
|
|
mcMMOPlayer.beginXpGain(skill, xp);
|
|
}
|
|
}
|